Sparkfunの”Qwiic – CCS811/BME280搭載 空気品質/環境センサモジュール”を入手したので、M5StickCを使って動作テストをしてみました。高度は気圧から算出しているようで、短時間でも1メートルぐらい上下するので参考にはなりません。関数を変えることで、気温は摂氏と華氏を、高度はメートルとフィートを切り替えることができます。
Characteristic | Range |
---|---|
tVOC(総揮発性有機化合物) | 0 – 1187 PPB |
eCO2(二酸化炭素濃度) | 400 – 8192 PPM |
Temperature(気温) | -40°C – 85°C |
Humidity(湿度) | 0 – 100% RH, ±3% from 20 – 80% |
Pressure(気圧) | 30 – 110 kPa, relative accuracy of 12 Pa, absolute accuracy of 100 Pa |
Altitude(高度) | 0 – 30,000ft (9.2km), relative accuracy of 3.3ft (1M) at sea level, 6.6ft (2M) at 30,000ft. |
M5StickC:https://www.switch-science.com/catalog/5517/
Qwiic – CCS811/BME280搭載 空気品質/環境センサモジュール:https://www.switch-science.com/catalog/3539/
Qwiicケーブル(Qwiic – 4ピンオス):https://www.switch-science.com/catalog/3541/
接続方法
Qwiicケーブルを使ってM5StickCのGPIOにつなぎました。
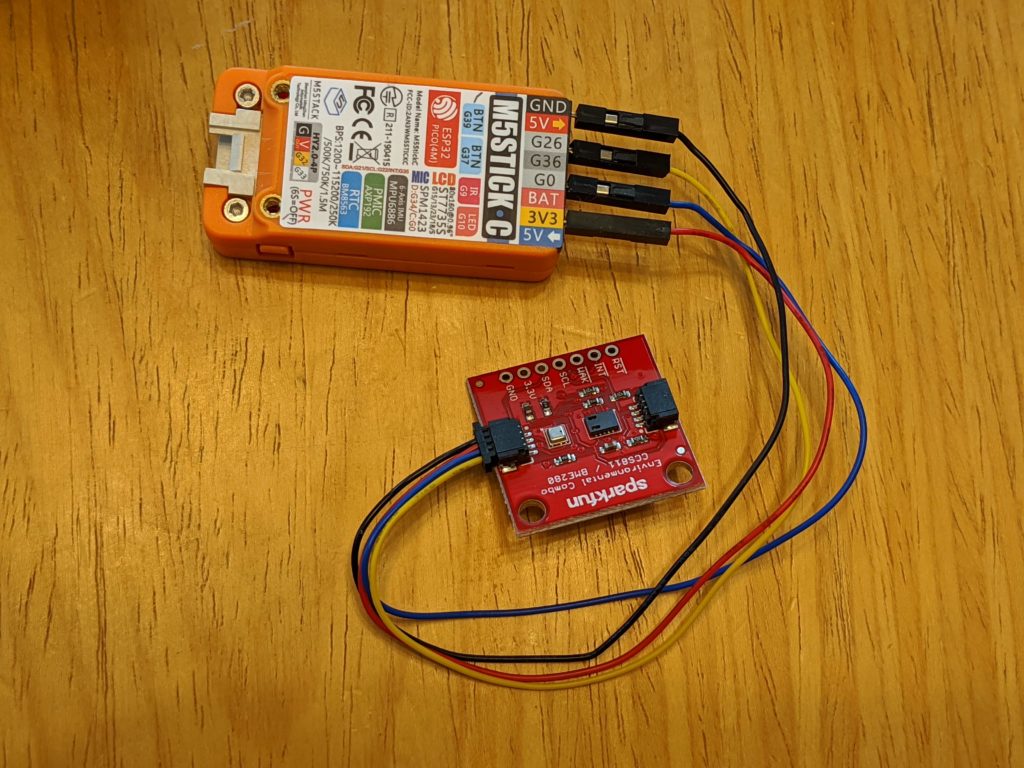
GND(黒):GMD
SDA(青):G0
SCL(黄):G26
電源(赤):3V3
GPIOの代わりにGROVE端子に繋いでも良いと思います。その場合はSDAがG32、SCLがG33に繋げばよいかと。
Arduinoのコード(シンプル版)
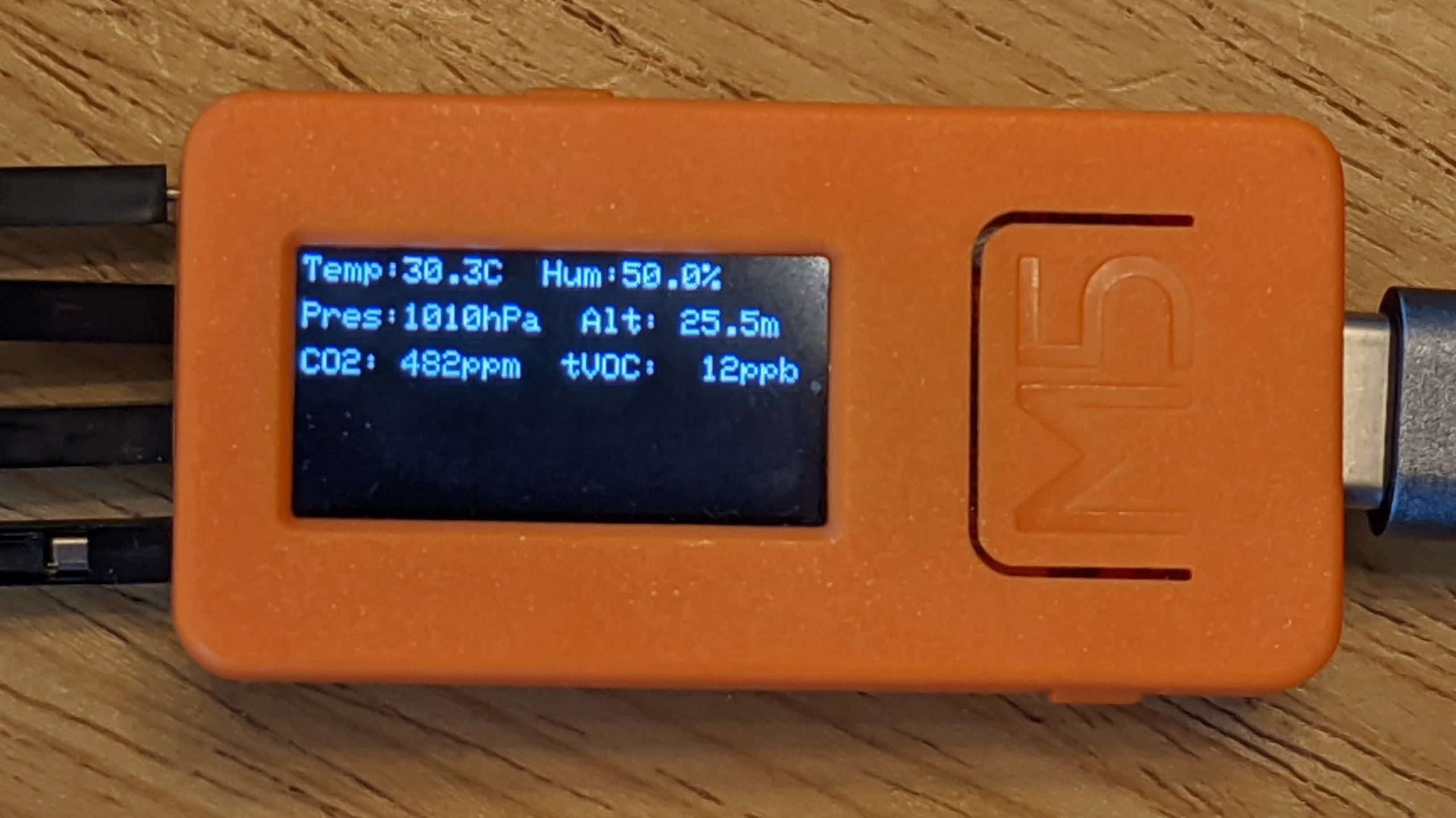
Arduinoのコードはサンプルコードをほぼほぼ流用
環境センサのライブラリは以下のアドレスから(真ん中の方にCCS811とBME280のライブラリのダウンロードリンクがあります)
https://learn.sparkfun.com/tutorials/ccs811bme280-qwiic-environmental-combo-breakout-hookup-guide
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 |
/* M5StickC with SparkFun Environmental Combo Breakout - CCS811/BME280 (Qwiic) 3.3V to 3.3V pin GND to GND pin SDA(Blue) to G0 or G32 SCL(Yellow) to G26 or G33 */ #include <M5StickC.h> #include <Wire.h> //Click here to get the library:https://learn.sparkfun.com/tutorials/ccs811bme280-qwiic-environmental-combo-breakout-hookup-guide?_ga=2.148422387.557098540.1597375634-463411088.1597375634 #include "SparkFunCCS811.h" #include "SparkFunBME280.h" CCS811 GasSensor(0x5B); BME280 TempSensor; void setup() { M5.begin(); M5.Lcd.setRotation(1); Serial.begin(115200); Serial.println("Environmental Combo Sensor Test"); Wire.begin(0, 26); if (GasSensor.begin() == false) { Serial.print("CCS811 error. Please check wiring. Freezing..."); while (1); //Freeze } if (TempSensor.beginI2C() == false) //Begin communication over I2C { Serial.println("The sensor did not respond. Please check wiring."); while(1); //Freeze } } void loop() { float humidity = TempSensor.readFloatHumidity(); float pressure = TempSensor.readFloatPressure(); float altitude = TempSensor.readFloatAltitudeMeters(); //float altitude = TempSensor.readFloatAltitudeFeet(); float temp = TempSensor.readTempC(); //float temp = TempSensor.readTempF(); int CO2, tVOC; //Check to see if data is ready with .dataAvailable() if (GasSensor.dataAvailable()) { //If so, have the sensor read and calculate the results. GasSensor.readAlgorithmResults(); CO2 = GasSensor.getCO2(); tVOC = GasSensor.getTVOC(); } M5.Lcd.fillScreen(BLACK); M5.Lcd.setTextColor(WHITE); M5.Lcd.setTextFont(1); M5.Lcd.setTextSize(1); //M5.Lcd.setTextSize(2); M5.Lcd.setCursor(4, 4); M5.Lcd.printf("Temp:%4.1fC Hum:%4.1f%%",temp, humidity); M5.Lcd.setCursor(4, 18); M5.Lcd.printf("Pres:%4.0fhPa Alt:%5.1fm",pressure/100, altitude); M5.Lcd.setCursor(4, 32); M5.Lcd.printf("CO2:%4dppm tVOC:%4dppb",CO2, tVOC); delay(200); } |
Arduinoのコード(カッコいい版)
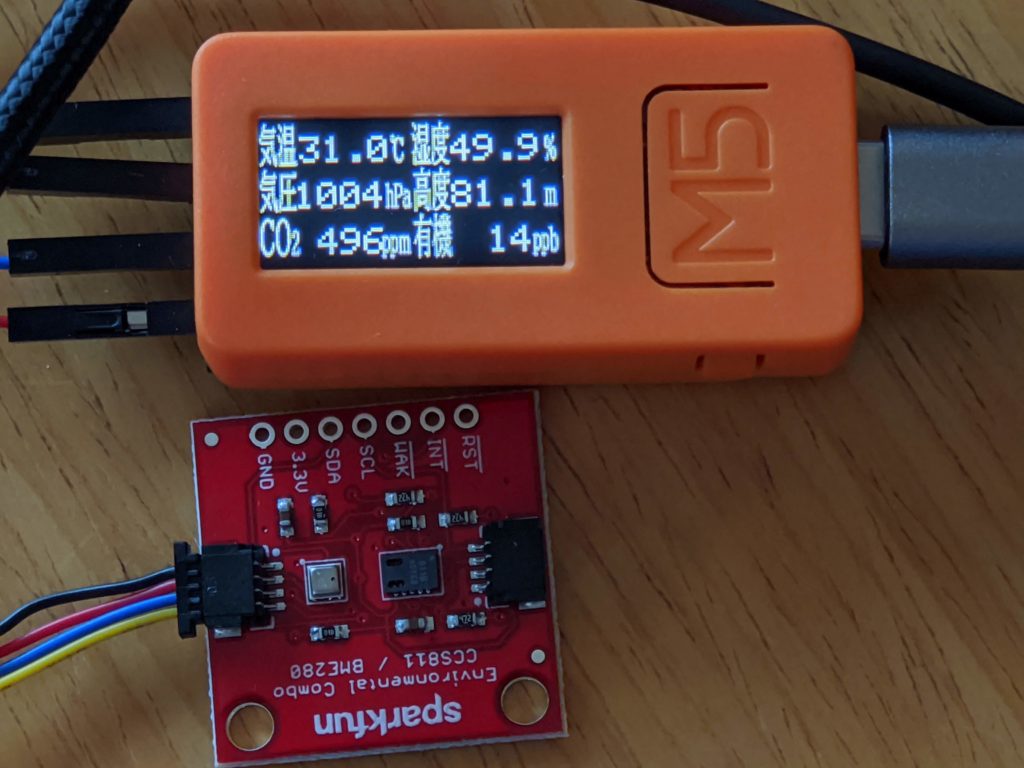
画像を使って、ちょっとカッコよくしてみた。
image.cをArduinoのプログラムファイルと同じフォルダ内に入れる必要があります。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 |
/* M5StickC with SparkFun Environmental Combo Breakout - CCS811/BME280 (Qwiic) 3.3V to 3.3V pin GND to GND pin SDA(Blue) to G0 or G32 SCL(Yellow) to G26 or G33 */ #include <M5StickC.h> #include <Wire.h> #include "image.c" //Click here to get the library:https://learn.sparkfun.com/tutorials/ccs811bme280-qwiic-environmental-combo-breakout-hookup-guide?_ga=2.148422387.557098540.1597375634-463411088.1597375634 #include "SparkFunCCS811.h" #include "SparkFunBME280.h" CCS811 GasSensor(0x5B); BME280 TempSensor; void setup() { M5.begin(); M5.Lcd.setRotation(1); Serial.begin(115200); Serial.println("Environmental Combo Sensor Test"); Wire.begin(0, 26); if (GasSensor.begin() == false) { Serial.print("CCS811 error. Please check wiring. Freezing..."); while (1); //Freeze } if (TempSensor.beginI2C() == false) //Begin communication over I2C { Serial.println("The sensor did not respond. Please check wiring."); while(1); //Freeze } } void loop() { float humidity = TempSensor.readFloatHumidity(); float pressure = TempSensor.readFloatPressure(); float altitude = TempSensor.readFloatAltitudeMeters(); //float altitude = TempSensor.readFloatAltitudeFeet(); float temp = TempSensor.readTempC(); //float temp = TempSensor.readTempF(); int CO2, tVOC; //Check to see if data is ready with .dataAvailable() if (GasSensor.dataAvailable()) { //If so, have the sensor read and calculate the results. //Get them later GasSensor.readAlgorithmResults(); CO2 = GasSensor.getCO2(); tVOC = GasSensor.getTVOC(); } //M5.Lcd.fillScreen(BLACK); M5.Lcd.drawBitmap(0, 0, 160, 80, img); M5.Lcd.setTextColor(WHITE); M5.Lcd.setTextFont(1); //M5.Lcd.setTextSize(1); M5.Lcd.setTextSize(2); /* //M5.Lcd.setCursor(4, 4); //M5.Lcd.printf("Temp:%4.1fC Hum:%4.1f%%",temp, humidity); M5.Lcd.setCursor(4, 18); //M5.Lcd.printf("Pres:%4.0fhPa Alt:%5.1fm",pressure/100, altitude); M5.Lcd.setCursor(4, 32); //M5.Lcd.printf("CO2:%4dppm tVOC:%4dppb",CO2, tVOC); */ M5.Lcd.setCursor(23, 10); M5.Lcd.printf("%4.1f",temp); M5.Lcd.setCursor(103, 10); M5.Lcd.printf("%4.1f",humidity); M5.Lcd.setCursor(20, 35); M5.Lcd.printf("%4.0f",pressure/100); M5.Lcd.setCursor(103, 35); M5.Lcd.printf("%4.1f",altitude); M5.Lcd.setCursor(20, 60); M5.Lcd.printf("%4d",CO2); M5.Lcd.setCursor(98, 60); M5.Lcd.printf("%4d",tVOC); delay(200); } |
コメント